When working with relational databases, data is often spread across multiple tables. To retrieve meaningful insights, we need to combine this data. This is where joins come into play. MySQL offers a variety of join types to combine rows from two or more tables based on related columns. Mastering these joins is essential for efficient data retrieval and query optimization.
In this blog post, we’ll explore the different types of joins available in MySQL, break down their syntax, and walk through practical examples using sample datasets. We’ll also cover when to use each type of join and provide tips to avoid common pitfalls.
What is a SQL Join?
A join in SQL is a method to combine rows from two or more tables based on a related column. The related column is usually a foreign key, but it can be any column that both tables have in common.
For example, consider two tables: employees
and departments
. The employees
table may have a column called department_id
that relates to the id
column in the departments
table. By using a join, you can combine data from both tables to get information about employees along with the department they belong to.
Types of Joins in MySQL
MySQL supports several types of joins, each with different behavior when matching rows between tables:
- INNER JOIN: Retrieves rows that have matching values in both tables.
- LEFT JOIN (or LEFT OUTER JOIN): Returns all rows from the left table and the matched rows from the right table. Unmatched rows in the right table will return NULL.
- RIGHT JOIN (or RIGHT OUTER JOIN): Returns all rows from the right table and the matched rows from the left table. Unmatched rows in the left table will return NULL.
- FULL OUTER JOIN: Retrieves rows when there is a match in either table. Unmatched rows from both tables will show NULL for columns from the other table. (Note: MySQL does not directly support FULL OUTER JOIN but can be simulated.)
- CROSS JOIN: Produces a Cartesian product of the two tables, returning all possible combinations of rows.
Let’s dive deeper into each of these join types with examples.
Example Setup: Dummy Data
To illustrate the different types of joins, let’s create two sample tables: employees
and departments
. The employees
table contains information about employees, while the departments
table contains information about the departments they work in.
-- Create departments table
CREATE TABLE departments (
id INT PRIMARY KEY,
department_name VARCHAR(50)
);
-- Insert sample data into departments
INSERT INTO departments (id, department_name) VALUES
(1, 'Human Resources'),
(2, 'Sales'),
(3, 'Engineering'),
(4, 'Marketing');
-- Create employees table
CREATE TABLE employees (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50),
department_id INT,
FOREIGN KEY (department_id) REFERENCES departments(id)
);
-- Insert sample data into employees
INSERT INTO employees (name, department_id) VALUES
('John Doe', 2),
('Jane Smith', 3),
('Samuel Green', 2),
('Diana Ross', NULL),
('Michael Brown', 1);
Now that we have our data set up, we can explore the different types of joins.
INNER JOIN
An INNER JOIN returns only the rows where there is a match in both tables. If an employee has no matching department, that employee will not appear in the result.
SELECT employees.name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.id;
Output:
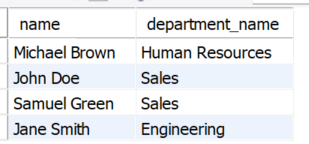
In this query, only employees who have a matching department_id
in the departments
table are returned. Notice that Diana Ross
, who has a NULL department_id
, is not included in the result.
LEFT JOIN (LEFT OUTER JOIN)
A LEFT JOIN returns all rows from the left table (employees
), even if there is no match in the right table (departments
). Unmatched rows from the right table are filled with NULL.
SELECT employees.name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.id;
Output:
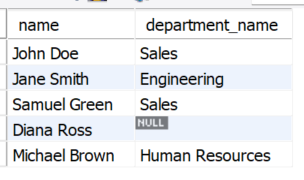
Here, all employees are listed, even if they don’t have a corresponding department. For Diana Ross
, the department_name
is NULL since her department_id
is NULL.
RIGHT JOIN (RIGHT OUTER JOIN)
A RIGHT JOIN returns all rows from the right table (departments
), even if there is no match in the left table (employees
). Unmatched rows from the left table are filled with NULL.
SELECT employees.name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.id;
Output:
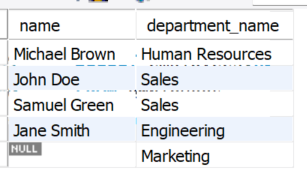
In this case, all departments are listed, including Marketing
, which has no employees. For this department, the name
column is NULL since there is no matching employee.
FULL OUTER JOIN (Simulated in MySQL)
MySQL doesn’t support a FULL OUTER JOIN directly, but you can simulate it using a combination of LEFT JOIN
and RIGHT JOIN
with a UNION
of the results.
SELECT employees.name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.id
UNION
SELECT employees.name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.id;
Output:
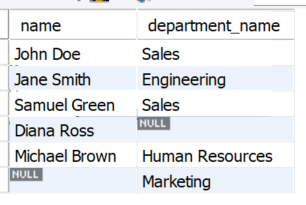
This query simulates a FULL OUTER JOIN by combining the results of a LEFT JOIN and a RIGHT JOIN, ensuring all rows from both tables are included.
CROSS JOIN
A CROSS JOIN returns the Cartesian product of two tables. This means that every row from the first table is combined with every row from the second table, resulting in a very large dataset.
SELECT employees.name, departments.department_name
FROM employees
CROSS JOIN departments;
Output:
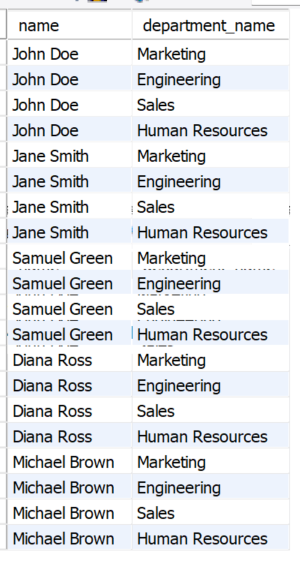
The result contains every possible combination of rows from the employees
and departments
tables. This type of join is rarely used but can be useful in specific cases like generating test data or performing matrix-style calculations.
When to Use Each Type of Join
- INNER JOIN: Use when you only want to return rows that have matching data in both tables.
- LEFT JOIN: Use when you want all rows from the left table, regardless of whether there’s a match in the right table.
- RIGHT JOIN: Use when you want all rows from the right table, regardless of whether there’s a match in the left table.
- FULL OUTER JOIN: Use when you want all rows from both tables, showing NULL where there is no match.
- CROSS JOIN: Use when you need a Cartesian product of the two tables.
Common Pitfalls When Using Joins
- Unintended Cartesian Products: Be careful when using
CROSS JOIN
or missing join conditions, as this can lead to very large and inefficient results. - Join on Non-Indexed Columns: Always try to join on columns that are indexed, as this will significantly improve performance. For example, ensure that
department_id
in theemployees
table is indexed if it’s frequently used in joins. - NULL Handling: Be mindful of NULL values, especially when using
LEFT JOIN
orRIGHT JOIN
, as they can affect the logic of your queries.
Conclusion
Understanding and using joins in MySQL is a critical skill for any developer or database administrator. Joins allow you to combine data from multiple tables, giving you the power to create complex queries that retrieve rich, meaningful insights from relational databases. By mastering the different types.